批量修改多台主机的SSH端口
脚本如下
import paramiko
import re
# 设置要修改的新SSH端口号
NEW_SSH_PORT = 2222
# 设置要修改SSH端口的主机信息
HOSTS = [
{'hostname': '10.0.0.1', 'username': 'root', 'password': 'your_password'},
{'hostname': '10.0.0.2', 'username': 'root', 'key_file': '/path/to/your/key_file'},
# 添加更多主机
]
# SSH配置文件路径
SSH_CONFIG_FILE = '/etc/ssh/sshd_config'
def modify_ssh_port(client, new_port):
"""修改SSH端口号"""
# 读取SSH配置文件内容
stdin, stdout, stderr = client.exec_command(f'cat {SSH_CONFIG_FILE}')
ssh_config = stdout.read().decode()
# 使用正则表达式替换端口号
new_config = re.sub(r'#?\s*Port\s+\d+', f'Port {new_port}', ssh_config)
# 备份原始配置文件
stdin, stdout, stderr = client.exec_command(f'cp {SSH_CONFIG_FILE} {SSH_CONFIG_FILE}.bak')
# 写入新的配置文件内容
stdin, stdout, stderr = client.exec_command(f'echo "{new_config}" > {SSH_CONFIG_FILE}')
# 重启SSH服务
stdin, stdout, stderr = client.exec_command('systemctl restart sshd')
print(f'主机 {client.get_transport().getpeername()[0]} 的SSH端口已修改为 {new_port}')
def main():
for host in HOSTS:
try:
client = paramiko.SSHClient()
client.set_missing_host_key_policy(paramiko.AutoAddPolicy())
if 'key_file' in host:
client.connect(hostname=host['hostname'], username=host['username'], key_filename=host['key_file'])
else:
client.connect(hostname=host['hostname'], username=host['username'], password=host['password'])
modify_ssh_port(client, NEW_SSH_PORT)
except Exception as e:
print(f'无法修改主机 {host["hostname"]} 的SSH端口: {e}')
finally:
client.close()
if __name__ == '__main__':
main()
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
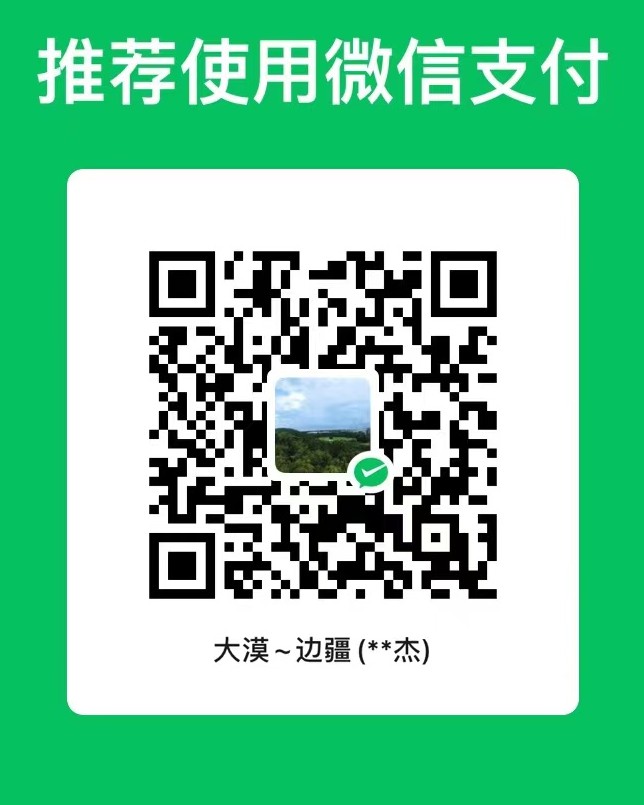
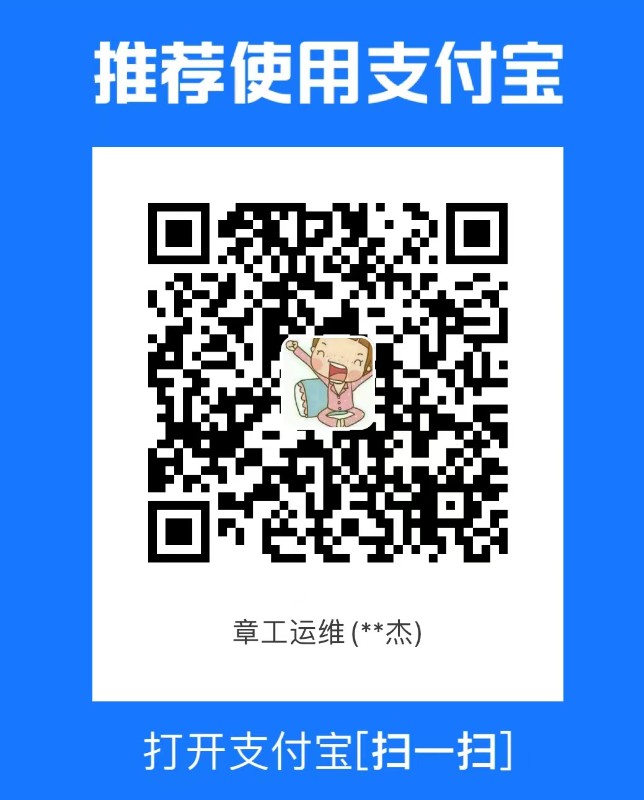
上次更新: 2024/06/12, 08:38:05